Successful Negotiations: A MasterClass
Learn to use all the related tools, walk into a job and be a rockstar from day one
Write your awesome label here.
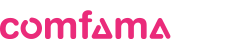
Derechos de autor © .
ComfamaPro
Ayuda y soporte

¡Puedes pagar por otros
medios de pago!
Pero recuerda
-
Puedes acceder 12 horas después del pago
-
Sólo puedes comprar plan anual o semestral
-
Debes registrarte con el mismo correo que ingresaste a ComfamaPro
-
La tarifa puede cambiar de acuerdo a tu categoría
Write your awesome label here.
Empty space, drag to resize
Déjanos tus datos
y uno de nuestros consultores te
contactará
Empty space, drag to resize